1. 컨트롤러
2. 한글 깨짐 설정
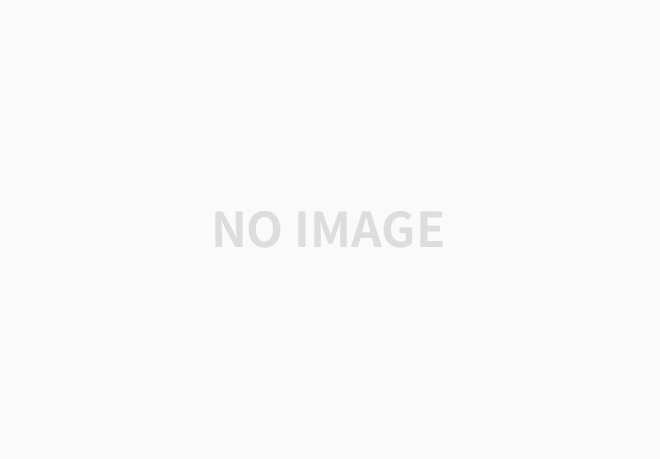
본 게시글은 Seoul wiz의 신입SW인력을 위한 실전 자바(Java) 스프링(Spring)을 참고하여 작성한 글입니다.
1. 컨트롤러
컨트롤러는 요청작업 후 뷰로 데이터 전달하는 역할을 한다.
@Controller로 클래스 생성 -> @RequestMapping으로 경로 지정 -> 처리 메소드 구현 -> 뷰 이름 리턴
한번 컨트롤러를 만들어보자. HomeController의 위치 옆에 SampleController.java를 만들었다.
@Controller
public class SampleController {
@RequestMapping("/sample") //요청 경로
public String samplecontent(Model model) {
//데이터 넣기
model.addAttribute("id", "birdmissile");
model.addAttribute("nickname", "howtolivelikehuman");
return "sample/samplecontent"; //뷰 이름
}
}
여기서 Model은 컨트롤러에서 데이터를 옴길때 사용한다.
samplecontent 메소드는 /sample의 request를 처리하여 기본 뷰 경로에서 sample/samplecontent.jsp로 리턴한다.
samplecontent.jsp의 body부분에서 다음과 같이 처리하면
<body> <!--알아서 잘 꺼내서 씀-->
<p>samplecontent.jsp 입니다.</p>
<p>ID = ${id}</p>
<p>Nickname = ${nickname}</p>
</body>
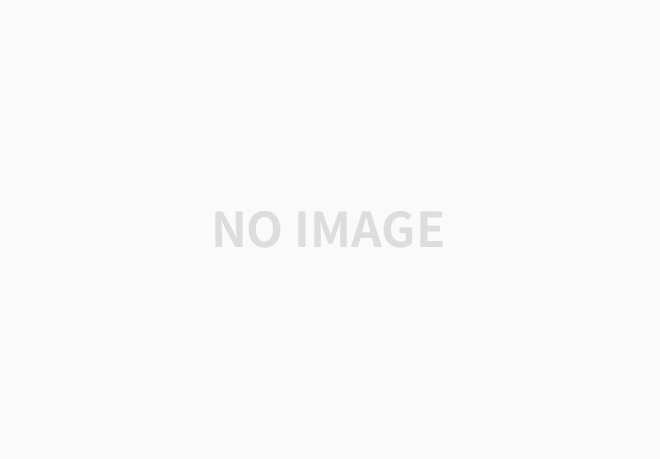
로직은 컨트롤러에서 처리하고, 최종적으로 맞는 뷰를 찾아갈 수 있도록 하는게 중요하다.
아니면 Model과 View를 혼합한 ModelAndView를 사용할 수도 있다.
@RequestMapping("/sample")
public ModelAndView samplecontent2() {
ModelAndView mav = new ModelAndView();
mav.addObject("id","birdmissile2");
mav.addObject("nickname","howtolivelikehuman2");
mav.setViewName("sample/samplecontent"); //다음 뷰
return mav;
}
아니면 아예 클래스에 RequestMapping을 적용할 수도 있다.
@Controller
@RequestMapping("/sample")
public class SampleController {
@RequestMapping("/content3")
public ModelAndView samplecontent3() {
ModelAndView mav = new ModelAndView();
mav.addObject("id","birdmissile2");
mav.addObject("nickname","howtolivelikehuman2");
mav.setViewName("sample/samplecontent");
return mav;
}
}
이렇게 한다면 /sample/content3의 request에서 samplecontent3이 실행되게 된다.
2. 한글 깨짐 설정
인코딩이 UTF-8이 아니라 한글 깨짐 현상이 발생할 수 있다.
우선 해결방법 하나는, JSP 페이지의 최상단에 enchoding을 포함한 정보를 명시하는 것이다.
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
html
다른 하나는 web.xml에 인코딩 필터를 설정하는 것이다.
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filer.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
html
'코딩 > 스프링 [JAVA]' 카테고리의 다른 글
Form 데이터 검증 [ Spring, Validator, @Valid, hibernate ] (0) | 2021.01.14 |
---|---|
Form 데이터 주고 받기 [Spring, HttpServletRequest] (0) | 2021.01.14 |
Spring MVC [Controller, servlet-context, eclipse] (1) | 2021.01.12 |
AOP (Aspect Oriented Programming) [Spring] (0) | 2021.01.11 |
외부 환경 파일 설정 (Environment, Property)[Spring] (0) | 2021.01.08 |
Comment